Introduction:
Welcome to the world of JavaScript! If you’re just starting with coding, you might wonder, “What exactly are data types?” Imagine you have a magic box where you can store all sorts of different things—numbers, words, even a simple yes or no answer. In programming, these “things” are called data types.
In this post, we’ll explore JavaScript’s seven primitive data types: String
, Number
, Boolean
, Null
, Undefined
, BigInt
, and Symbol
. By the end of this guide, you’ll understand what these types are, how they work, and why they are important for coding.
Let’s dive in!
1. What are Data Types in JavaScript?
Before we dive into JavaScript, let’s first understand what a data type is. Think of data types as different kinds of boxes that can hold different things. For example, in real life, you wouldn’t use a jewelry box to store a basketball, right? Similarly, in programming, data types define the type of data that can be stored and manipulated.
In JavaScript, data types help the program understand what kind of value you’re working with. Is it text? A number? A true/false condition? Each of these is a different data type.
Why Are Data Types Important?
Data types are crucial because they ensure that your program behaves correctly. If you mix up data types, your code might not work as expected, leading to errors and bugs. For instance, if you try to multiply a word by a number, JavaScript won’t know what to do!
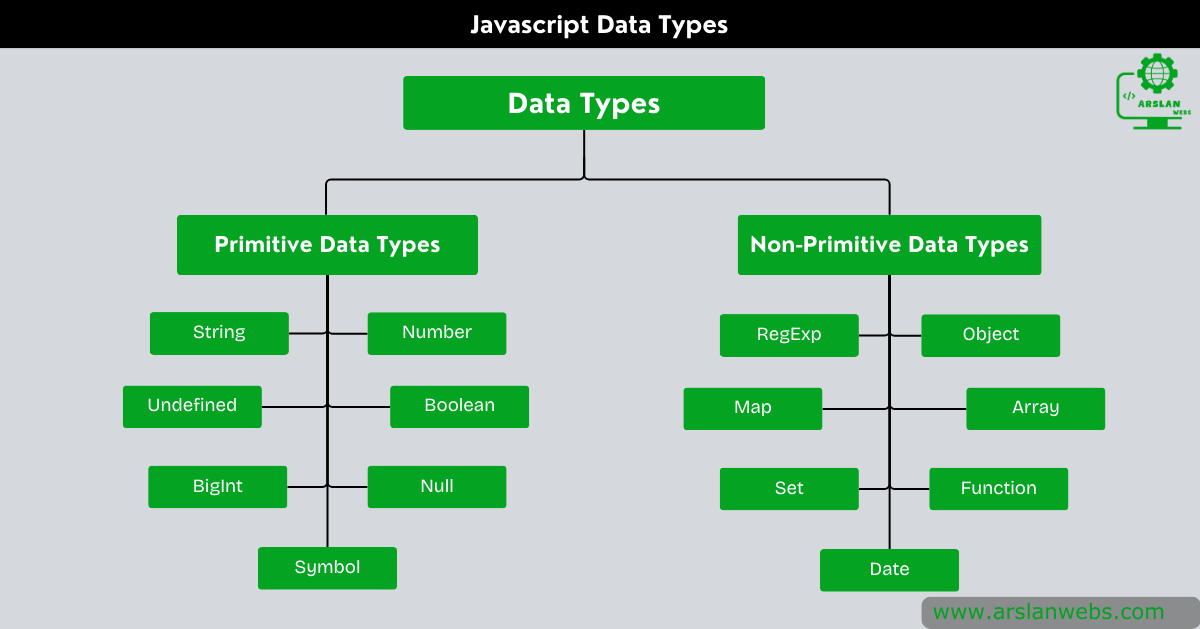
2. JavaScript Types are Dynamic
2.1. What Does “Dynamic” Mean?
In some programming languages, you must specify the data type when you create a variable, and it stays that way. However, JavaScript is more flexible. In JavaScript, variables are dynamic—this means you don’t have to specify a data type when you declare a variable. The type of the variable can change as the program runs.
let myData = "Hello, World!"; // Initially, myData is a String
myData = 42; // Now, myData is a Number
JavaScript2.2. Why Is This Flexibility Useful?
This flexibility makes JavaScript easier to learn and use, especially for beginners. You can focus on what your program needs to do without worrying too much about strict rules. However, it also means you have to be careful because it’s easy to make mistakes if you’re not sure what type of data you’re working with.
Placeholder for Image: A visual representation showing a variable changing types, e.g., from text to a number.
3. The Seven JavaScript Primitive Data Types
JavaScript has seven primitive data types, which are the simplest forms of data. These types are called “primitive” because they are basic and can’t be broken down into simpler parts.
3.1. String
A String is a sequence of characters used to represent text. You can think of a string as any text inside quotes. In JavaScript, you can use single quotes ('
), double quotes ("
), or backticks (`
) to define a string.
let name = "Alice"; // Double quotes
let greeting = 'Hello!'; // Single quotes
let message = `Hi, ${name}!`; // Backticks for template literals
JavaScriptStrings are incredibly useful for displaying text, handling user input, and many other tasks.
Key Characteristics:
- Strings can contain letters, numbers, symbols, and even emojis.
- Strings are immutable, meaning once created, they can’t be changed. You can only create a new string.
Placeholder for Image: An image of text in different quote styles ("Hello"
, 'World'
, `Hi`
).
3.2. Number
A Number in JavaScript can be an integer (a whole number) or a floating-point number (a number with a decimal point). Numbers are used for all kinds of calculations, from simple arithmetic to complex mathematical operations.
let age = 13; // An integer
let price = 19.99; // A floating-point number
JavaScriptJavaScript also has special numerical values like Infinity
, -Infinity
, and NaN
(which stands for “Not a Number”).
Key Characteristics:
- Numbers can be positive or negative.
- JavaScript uses a 64-bit floating-point format, which means it can represent a wide range of numbers.
Placeholder for Image: A diagram showing different types of numbers, including integers, floating points, and special values like NaN
.
3.3. Boolean
A Boolean represents one of two values: true
or false
. Booleans are often used in conditions to make decisions in your code.
let isStudent = true;
let hasGraduated = false;
JavaScriptBooleans are fundamental to controlling the flow of a program. For example, they can determine whether a certain block of code should run or not.
Key Characteristics:
- Booleans are the simplest data type and have only two possible values.
- They are often the result of comparisons or logical operations.
Placeholder for Image: An image showing a true/false switch or a yes/no toggle.
3.4. Null
Null is a special value in JavaScript that represents “nothing” or “no value.” It’s used to intentionally indicate the absence of any object value.
let emptyValue = null;
JavaScriptIt’s important to note that null
is different from 0
or an empty string (""
), which are values. Null
specifically means that a variable is empty or unknown.
Key Characteristics:
Null
is treated as a falsy value in JavaScript, meaning it’s consideredfalse
when evaluated in a Boolean context.- It’s often used to clear the value of a variable.
Placeholder for Image: An illustration of an empty box labeled “Null” to signify nothingness.
3.5. Undefined
Undefined is another special value in JavaScript. It indicates that a variable has been declared but hasn’t been assigned a value yet.
let notAssigned;
console.log(notAssigned); // Outputs: undefined
JavaScriptUnlike null
, which you can assign to a variable, undefined
is automatically assigned by JavaScript when you create a variable without initializing it.
Key Characteristics:
Undefined
is also a falsy value.- It’s different from
null
, which is an intentional absence of value, whileundefined
means no value has been assigned yet.
Placeholder for Image: A graphic showing a box labeled “Undefined” with a question mark inside, indicating no value.
3.6. BigInt
A BigInt is a special type of number that allows you to represent integers larger than the maximum safe integer (2^53 - 1
) that the Number type can handle. You can create a BigInt by adding an n
to the end of an integer.
let largeNumber = 123456789012345678901234567890n;
JavaScriptBigInt is useful for working with very large numbers that are beyond the range of the Number type.
Key Characteristics:
- BigInts are primarily used in areas like cryptography where very large numbers are common.
- BigInt cannot be mixed with Number types directly without converting one of them.
Placeholder for Image: A visual representation of a large number with an n
at the end to signify a BigInt.
3.7. Symbol
A Symbol is a unique and immutable primitive value that is often used to identify object properties. Symbols are created using the Symbol()
function.
let uniqueId = Symbol("id");
JavaScriptSymbols are particularly useful when you want to create a property that won’t accidentally conflict with other properties.
Key Characteristics:
- Every Symbol is unique, even if two Symbols are created with the same description.
- Symbols are not visible in for…in loops and are ignored by JSON.stringify.
Here’s a table of all the Primitive Data Types in JavaScript:
Primitive Data Type | Description | Example |
---|---|---|
String | Represents a sequence of characters (text). | "Hello" , 'World' , `Hi ${name}` |
Number | Represents numerical values, including integers and floating-point numbers. | 42 , 3.14 , -10 |
BigInt | Represents integers with arbitrary precision (for numbers larger than Number.MAX_SAFE_INTEGER ). | 12345678901234567890n , 9007199254740991n |
Boolean | Represents logical values: true or false . | true , false |
Undefined | Automatically assigned when a variable is declared but not initialized. | undefined |
Null | Represents an intentional absence of a value. | null |
Symbol | Represents a unique, immutable value, often used as object property keys. | Symbol('id') , Symbol() |
This table includes the six primary primitive data types along with examples and their basic descriptions.
4. Key Characteristics of Primitive Data Types
Now that you know about the different primitive data types in JavaScript, let’s look at some of their key characteristics:
- Immutability: Once a primitive value is created, it cannot be changed. For example, you can’t alter the characters in a string or the value of a number directly.
- Stored by Value: Primitive data types are stored by value, meaning each variable holds its own copy of the data. When you assign a primitive value to another variable, JavaScript creates a new copy.
- Automatic Conversion: JavaScript automatically converts between data types when necessary. For example, if you try to add a number to a string, JavaScript will convert the number to a string before performing the operation.
- Comparison: Primitive values are compared by value. For example, two strings are considered equal if they contain the same sequence of characters.
- Memory Efficiency: Since primitive types are simple and immutable, they are stored in a more memory-efficient way compared to complex objects.
Here’s a table of the Key Characteristics of Primitive Data Types in JavaScript:
Characteristic | Description | Example |
---|---|---|
Immutability | Primitive values cannot be changed once created. Modifying a primitive creates a new value. | let str = "hello"; str[0] = 'H'; // str is still "hello" |
Stored by Value | When assigned or passed to a function, a copy of the value is made (not a reference). | let x = 5; let y = x; x = 10; // y is still 5 |
Type and Value Comparison | Primitives are compared by their value and type when using strict equality (=== ). | 5 === '5'; // false |
Type Coercion | In loose equality (== ), JavaScript attempts to convert values to the same type before comparing. | 5 == '5'; // true |
Default Value Assignment | Variables declared but not initialized are automatically assigned undefined . | let a; console.log(a); // undefined |
Cannot Hold Complex Data | Primitive types hold only simple data (e.g., strings, numbers) and not collections or objects. | let age = 30; // primitive value |
Memory Efficiency | Primitive values are stored directly in memory, resulting in efficient and lightweight data handling. | let isValid = true; // stored directly by value |
This table outlines the core characteristics of how JavaScript handles primitive data types, with examples to illustrate each feature.
5. Why Understanding Data Types is Important
Understanding JavaScript primitive data types is important because it helps you write better and more efficient code. It allows you to:
- Avoid Bugs: Knowing the difference between
null
andundefined
can help prevent errors. - Optimize Performance: Proper use of types can make your code run faster.
- Improve Readability: Clear use of types makes your code easier to understand for others.
Placeholder for Image: An infographic summarizing the benefits of understanding data types.
6. Best Practices for Using Primitive Data Types
- Always Initialize Variables: Avoid using uninitialized variables to prevent
undefined
errors. - Use Descriptive Names: Use variable names that describe the data type or its purpose.
- Avoid Mixing Types: Try to avoid mixing types (like adding a string to a number) unless necessary.
- Be Aware of Type Coercion: JavaScript sometimes automatically converts types, so be aware of this behavior.
Conclusion
JavaScript primitive data types are the basic building blocks of any program you write in this language. By understanding these types—String, Number, Boolean, Null, Undefined, BigInt, and Symbol—you can start creating more dynamic and effective programs. Remember to use these types appropriately, follow best practices, and keep exploring how they work in different scenarios.
Now that you’ve learned about these fundamental concepts, try using them in your own projects and see how they make your code more powerful!